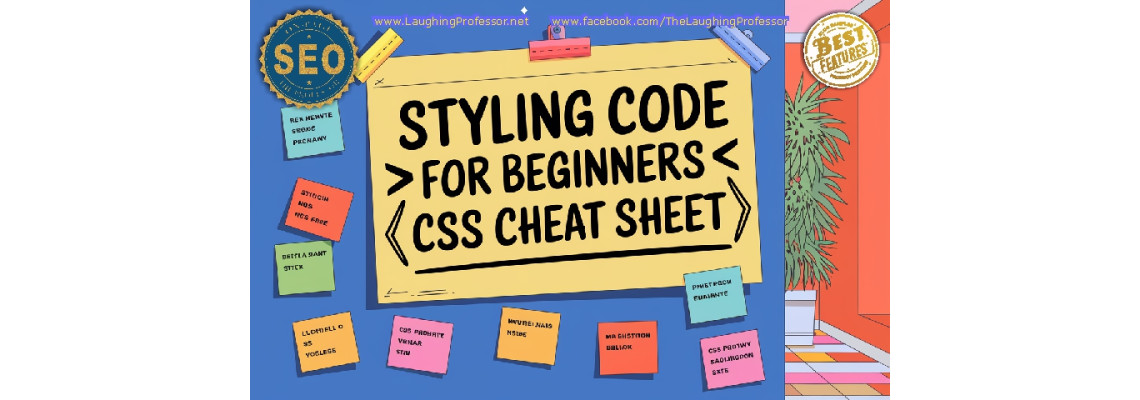
Basic Styling code for beginners.
<style>
CSS 'Cheat' Sheet. </style>
CSS is fantastic for visual styling, appearance within a web page of any subject matter such as our 'SEO Services comparison chart'. CSS can be written in the <body> tags, but because it can be lengthy and slow down your page loading speed, a separate file is created just for the styling code and a meta link placed in the main page header. This can be called "anything.css"
and you can have more than one, if "needed.css"
and place in the same folder as the page using it.
<head>
<link rel="stylesheet" href="styles.css">
</head>
<style>
#anything {
width: 100px;
height: 50px
;
}
.something-else {
padding: 10px 20px;
}
</style> is the same as:
<style>#anything {width: 100px;height: 50px ;} .something-else {padding: 10px 20px;}</style>
1. Box Model Properties
width
,height
: Defines the size of an element.Example: This sets the width to 100 pixels and the height to 50 pixels.
padding
: Space between the content and the border.Example: Adds 10px padding to the top/bottom and 20px to the left/right.
margin
: Space outside the border (between elements).Example: Adds 15px margin on all sides.
border
: Defines the border style.Example: Creates a red border that is 2 pixels thick and solid.
box-sizing
: Defines how width and height are calculated (whether padding and border are included).Example: Ensures that padding and borders are included in the element’s width and height.
2. Background and Colors
background-color
: Sets the background color.Example: Fills the background with a yellow color.
background-image
: Adds an image as the background.Example: Displays the image
image.jpg
as the background.background-size
: Resizes the background image.Example: Ensures the background image covers the entire element without stretching.
background-position
: Controls the position of the background image.Example: Positions the background image in the center of the element.
background-repeat
: Controls if the background image repeats.Example: Prevents the background image from repeating.
3. Borders and Outlines
border
: Defines the border style, thickness, and color.Example: Creates a green, dashed border that is 2px thick.
border-radius
: Rounds the corners of an element.Example: Adds 10px rounding to each corner.
outline
: Similar to border, but does not affect layout.Example: Creates a blue, dotted outline that’s 3px wide.
outline-offset
: Adds space between the border and the outline.Example: Adds a 5px space between the border and the outline.
4. Shadows
box-shadow
: Adds shadow around the element.Example: Adds a shadow that is 5px to the right, 5px down, blurred by 10px, and has 50% opacity (black shadow).
text-shadow
: Adds shadow to text.Example: Adds a shadow that is offset by 2px horizontally and vertically, blurred by 4px, and is black.
Explanation:
2px
(horizontal offset),2px
(vertical offset),4px
(blur radius),#000
(color of shadow).
5. Text and Typography
color
: Sets the text color.Example: Sets the text color to a dark gray.
font-size
: Defines the size of the font.Example: Sets the font size to 16 pixels.
font-weight
: Defines the thickness of the font.Example: Makes the text bold.
font-style
: Defines the style of the font.Example: Makes the text italicized.
text-align
: Aligns text horizontally.Example: Centers the text within the element.
text-transform
: Changes the case of text.Example: Transforms all text to uppercase.
line-height
: Defines the spacing between lines of text.Example: Sets the line spacing to 1.5 times the font size.
letter-spacing
: Adjusts the space between letters.Example: Adds 2px of space between each letter.
6. Positioning and Display
position
: Controls how an element is positioned on the page.Example: Places the element 50px from the top and 100px from the left of the nearest positioned ancestor.
z-index
: Controls the stacking order of elements.Example: Ensures the element is stacked above elements with a lower
z-index
.display
: Defines how the element behaves in the layout.Example: Makes the element a flex container, enabling flexbox layout.
visibility
: Toggles element visibility without affecting layout.Example: Hides the element but still occupies space on the page.
7. Flexbox Layout
justify-content
: Aligns flex items along the main axis.Example: Distributes items evenly with space between them.
align-items
: Aligns flex items along the cross axis.Example: Vertically centers flex items within the container.
8. Grid Layout
grid-template-columns
: Defines the number and size of grid columns.Example: Creates a grid with two columns, where the second is twice as wide as the first.
grid-gap
: Defines the spacing between grid items.Example: Adds 10px of space between each grid item.
9. Transitions and Animations
transition
: Smoothly changes CSS properties over time.Example: Any property change will take 0.3 seconds and follow an easing function.
animation
: Creates CSS animations.Example: Animates the element with the
move
keyframe for 5 seconds and repeats indefinitely.Define the keyframe:
10. Transforms
transform
: Applies 2D or 3D transformations.Example: Scales the element to 1.5 times its original size.
Example: Rotates the element 45 degrees.
11. Hover and Focus States
:hover
: Applies styles when an element is hovered.Example: Changes the background color of a button to yellow when hovered.
:focus
: Applies styles when an element is focused.Example: Changes the border color to blue when an input field is focused.
12. Cursor and User Interaction
cursor
: Changes the mouse cursor when hovering over the element.Example: Changes the cursor to a pointer when hovered.
13. Overflow and Scrolling
overflow
: Controls how content that overflows the element’s box is handled.Example: Adds scroll bars when the content overflows the container.
14. Filter and Effects
filter
: Adds visual effects to elements.Example: Applies a 5px blur to the element.
Example: Increases the brightness by 150%.
opacity
: Controls the transparency of the element.Example: Makes the element 50% transparent.
Leave a Comment